توضیحات :
سورس کد پیاده سازی برنامه ماشین حساب پیشرفته (ماشین حساب مهندسی) در JAVA
همراه با فایلهای .jar , .java و سایر فایلهای لازم
بخشهایی از سورس کد این پروژه :
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Container;
import java.awt.Cursor;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.GridLayout;
import java.awt.Insets;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.io.IOException;
import javax.swing.ButtonGroup;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.JTextField;
import javax.swing.UIManager;
import javax.swing.WindowConstants;
import javax.swing.border.EmptyBorder;
public class Calc extends JFrame implements ActionListener,MouseListener //,KeyListener
{
private JButton jb_one,jb_two,jb_three,jb_four,jb_five,jb_six,jb_seven,jb_eight,jb_nine,jb_zero;
private JButton jb_plus,jb_minus,jb_divide,jb_multiply;
private JButton jb_sin,jb_cos,jb_tan,jb_asin,jb_acos,jb_atan,jb_pie,jb_E;
private JButton jb_decimalpoint,jb_equalto,jb_fact,jb_power,jb_changesign,jb_reciporcal;
private JButton jb_todeg,jb_torad,jb_round,jb_CA,jb_CE,jb_Backspace;
private JRadioButton jrb_deg,jrb_rad;
private ButtonGroup jrb_group;
private JTextField jtf_display;
private JLabel jl_url;
private double tempdisplayNum;
boolean plusPressed,minusPressed,mulPressed,divPressed,equalPressed,powerPressed;
/**
* This constructor forms GUI and add required Compontents
* to listeners.
*/
public Calc()
{
super(“Scientic Calculator ( Currently running on JVM Version ” + System.getProperty(“java.version”) + ” )”);
/**
* Sets ImageIcon to the Title of the Frame
* @param ImageIcon
*/
setIconImage(Toolkit.getDefaultToolkit().getImage(Calc.class.getResource(“calc.gif”)));
/*
* We don’t want window to close automatically by default
*/
setDefaultCloseOperation(WindowConstants.DO_NOTHING_ON_CLOSE);
/**
* Inner Class for Main Window Listener.It is executed when
* window is closing.It cleans the memmory explictly and then it
* application exits.
*/
addWindowListener(new WindowAdapter()
{
public void windowClosing(WindowEvent e)
{
Calc.this.dispose();
System.runFinalization();
System.gc();
System.exit(0);
}
});
tempdisplayNum = 0;
resetAllButton();
equalPressed = false;
/*
* GUI Formation
*/
JPanel jp_main = new JPanel();
jp_main.setLayout(new BorderLayout());
jp_main.setBorder(new EmptyBorder(new Insets(3,5,5,5)));
JPanel jp_top = new JPanel();
jp_top.setLayout(new BorderLayout());
JPanel jp_top_down = new JPanel();
jp_top_down.setLayout(new BorderLayout());
JPanel jp_top_west = new JPanel();
jb_Backspace = new JButton(“BackSpace”);
jp_top_west.setLayout(new FlowLayout(FlowLayout.LEFT,0,5));
jp_top_west.add(jb_Backspace);
JPanel jp_top_east = new JPanel();
jp_top_east.setLayout(new FlowLayout(FlowLayout.RIGHT));
jtf_display = new JTextField();
jtf_display.setEditable(false);
jtf_display.setHorizontalAlignment( JTextField.RIGHT );
// jtf_display.setFocusable(false);
jtf_display.setRequestFocusEnabled(false);
jtf_display.setBackground(Color.white);
jrb_deg = new JRadioButton(“Degrees”);
jrb_rad = new JRadioButton(“Radian”);
jrb_deg.setSelected(true);
jrb_group = new ButtonGroup();
jrb_group.add(jrb_deg);
jrb_group.add(jrb_rad);
jp_top_east.add(jrb_deg);
jp_top_east.add(jrb_rad);
jp_top_down.add(jp_top_east,BorderLayout.EAST);
jp_top_down.add(jp_top_west,BorderLayout.WEST);
jp_top.setLayout(new BorderLayout());
JPanel jp_top_top = new JPanel();
jp_top_top.setLayout(new BorderLayout());
jl_url = new JLabel(“Rajesh’s homepage”);
jl_url.setForeground(Color.blue);
jl_url.addMouseListener(Calc.this);
jp_top_top.add(new JLabel(“This Calculator is created by Rajesh.”),BorderLayout.WEST);
jp_top_top.add(jl_url,BorderLayout.EAST);
jp_top.add(jp_top_top,BorderLayout.NORTH);
jp_top.add(jtf_display,BorderLayout.CENTER);
jp_top.add(jp_top_down,BorderLayout.SOUTH);
JPanel jp_center = new JPanel();
jp_center.setLayout(new GridLayout(5,7,5,5));
jb_one = new JButton(“1”);
jb_two = new JButton(“2”);
jb_three = new JButton(“3”);
jb_four = new JButton(“4”);
jb_five = new JButton(“5”);
jb_six = new JButton(“6”);
jb_seven = new JButton(“7”);
jb_eight = new JButton(“8”);
jb_nine = new JButton(“9”);
jb_zero = new JButton(“0”);
jb_plus = new JButton(“+”);
jb_minus = new JButton(“-“);
jb_divide = new JButton(“/”);
jb_multiply = new JButton(“*”);
jb_sin = new JButton(“Sin”);
jb_cos = new JButton(“Cos”);
jb_tan = new JButton(“Tan”);
jb_asin = new JButton(“aSin”);
jb_acos = new JButton(“aCos”);
jb_atan = new JButton(“aTan”);
jb_pie = new JButton(“PI”);
jb_E = new JButton(“E”);
jb_decimalpoint = new JButton(“.”);
jb_equalto = new JButton(“=”);
jb_fact = new JButton(“x!”);
jb_power = new JButton(“x^n”);
jb_changesign = new JButton(“+/-“);
jb_reciporcal = new JButton(“1/x”);
jb_todeg = new JButton(“toDeg”);
jb_torad = new JButton(“toRad”);
jb_round = new JButton(“Round”);
jb_CA = new JButton(“CA”);
jb_CE = new JButton(“CE”);
/**
* Adding Button to Listeners
*/
jb_one.addActionListener(Calc.this);
jb_two.addActionListener(Calc.this);
jb_three.addActionListener(Calc.this);
jb_four.addActionListener(Calc.this);
jb_five.addActionListener(Calc.this);
jb_six.addActionListener(Calc.this);
jb_seven.addActionListener(Calc.this);
jb_eight.addActionListener(Calc.this);
jb_nine.addActionListener(Calc.this);
jb_zero.addActionListener(Calc.this);
jb_plus.addActionListener(Calc.this);
jb_minus.addActionListener(Calc.this);
jb_divide.addActionListener(Calc.this);
jb_multiply.addActionListener(Calc.this);
jb_sin.addActionListener(Calc.this);
jb_cos.addActionListener(Calc.this);
jb_tan.addActionListener(Calc.this);
jb_asin.addActionListener(Calc.this);
jb_acos.addActionListener(Calc.this);
jb_atan.addActionListener(Calc.this);
jb_pie.addActionListener(Calc.this);
jb_E.addActionListener(Calc.this);
jb_decimalpoint.addActionListener(Calc.this);
jb_equalto.addActionListener(Calc.this);
jb_fact.addActionListener(Calc.this);
jb_power.addActionListener(Calc.this);
jb_changesign.addActionListener(Calc.this);
jb_reciporcal.addActionListener(Calc.this);
jb_todeg.addActionListener(Calc.this);
jb_torad.addActionListener(Calc.this);
jb_round.addActionListener(Calc.this);
jb_CA.addActionListener(Calc.this);
jb_CE.addActionListener(Calc.this);
jb_Backspace.addActionListener(Calc.this);
/*
jb_one.addKeyListener(Calc.this);
jb_one.addKeyListener(Calc.this);
jb_two.addKeyListener(Calc.this);
jb_three.addKeyListener(Calc.this);
jb_four.addKeyListener(Calc.this);
jb_five.addKeyListener(Calc.this);
jb_six.addKeyListener(Calc.this);
jb_seven.addKeyListener(Calc.this);
jb_eight.addKeyListener(Calc.this);
jb_nine.addKeyListener(Calc.this);
jb_zero.addKeyListener(Calc.this);
jb_plus.addKeyListener(Calc.this);
jb_minus.addKeyListener(Calc.this);
jb_divide.addKeyListener(Calc.this);
jb_multiply.addKeyListener(Calc.this);
jb_sin.addKeyListener(Calc.this);
jb_cos.addKeyListener(Calc.this);
jb_tan.addKeyListener(Calc.this);
jb_asin.addKeyListener(Calc.this);
jb_acos.addKeyListener(Calc.this);
jb_atan.addKeyListener(Calc.this);
jb_pie.addKeyListener(Calc.this);
jb_E.addKeyListener(Calc.this);
jb_decimalpoint.addKeyListener(Calc.this);
jb_equalto.addKeyListener(Calc.this);
jb_fact.addKeyListener(Calc.this);
jb_power.addKeyListener(Calc.this);
jb_changesign.addKeyListener(Calc.this);
jb_reciporcal.addKeyListener(Calc.this);
jb_todeg.addKeyListener(Calc.this);
jb_torad.addKeyListener(Calc.this);
jb_round.addKeyListener(Calc.this);
jb_CA.addKeyListener(Calc.this);
jb_CE.addKeyListener(Calc.this);
jb_Backspace.addKeyListener(Calc.this);
*/
jp_center.add(jb_one);
jp_center.add(jb_two);
jp_center.add(jb_three);
jp_center.add(jb_multiply);
jp_center.add(jb_reciporcal);
jp_center.add(jb_sin);
jp_center.add(jb_asin);
jp_center.add(jb_four);
jp_center.add(jb_five);
jp_center.add(jb_six);
jp_center.add(jb_divide);
jp_center.add(jb_power);
jp_center.add(jb_cos);
jp_center.add(jb_acos);
jp_center.add(jb_seven);
jp_center.add(jb_eight);
jp_center.add(jb_nine);
jp_center.add(jb_plus);
jp_center.add(jb_changesign);
jp_center.add(jb_tan);
jp_center.add(jb_atan);
jp_center.add(jb_zero);
jp_center.add(jb_decimalpoint);
jp_center.add(jb_equalto);
jp_center.add(jb_minus);
jp_center.add(jb_fact);
jp_center.add(jb_pie);
jp_center.add(jb_E);
jp_center.add(jb_CA);
jp_center.add(jb_CE);
jp_center.add(jb_round);
jp_center.add(jb_todeg);
jp_center.add(jb_torad);
Container cp = this.getContentPane();
jp_main.add(jp_top,BorderLayout.NORTH);
jp_main.add(jp_center,BorderLayout.CENTER);
cp.add(jp_main,BorderLayout.CENTER);
setSize(520,250);
/* Packing all Commponent, so spaces are left */
pack();
/* Making Window to appear in Center of Screen */
Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize();
Dimension frameSize = this.getPreferredSize();
setLocation(screenSize.width/2 – (frameSize.width/2),
screenSize.height/2 – (frameSize.height/2));
setVisible(true);
this.requestFocus();
}
تصاویری از محیط این برنامه :
=================================
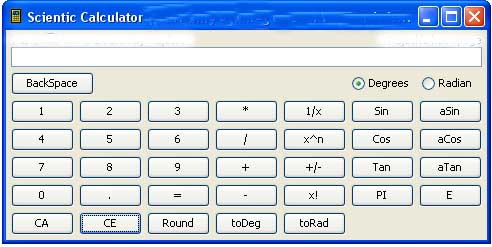
|
دیدگاهتان را بنویسید